An Experiment in Instagram Automation
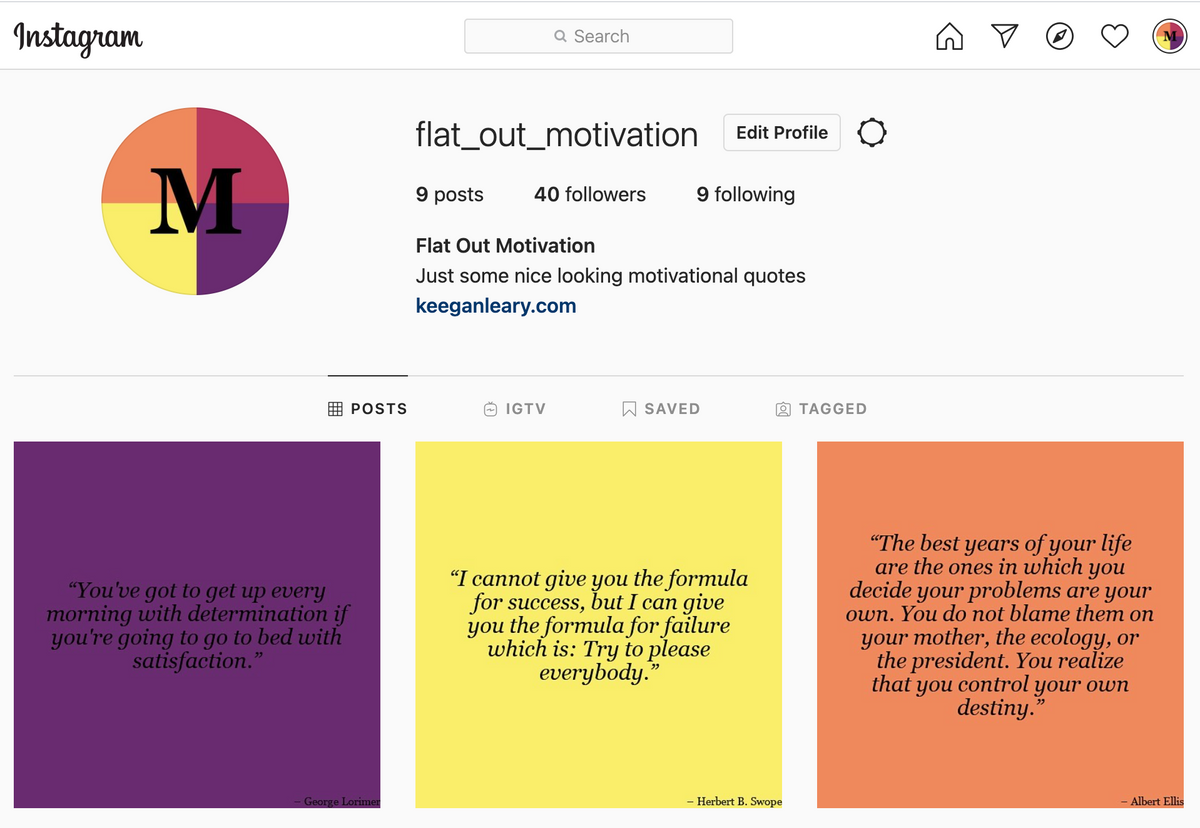
What if you could automatically generate content on Instagram and become famous on that platform without actually doing anything? Sounds interesting so I figured I would give it a try.
In my journey to learn to code, I've been working with python libraries PIL and Tkinter which are designed to build User Interfaces or work with images on screen. I want to work a little more with these libraries to solidify my learning. It also seems that you can't escape motivational quotes these days on IG.
"I am my own roadblock."
"The greatest risk is not taking one."
"Strive for progress not perfection."
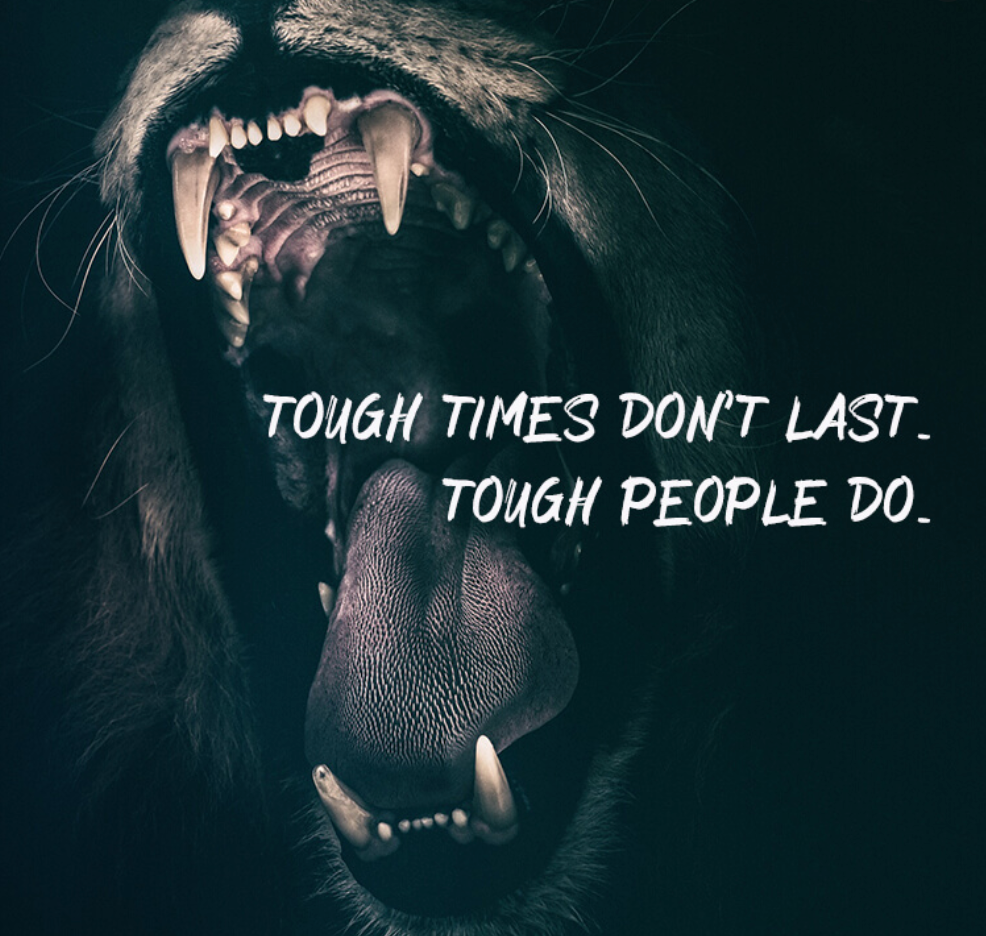
OK I figured any schmuck can take a stock photo of a lion or a lambo and put some words on top of it. But have I learned enough to make python do it for me, automatically?
I also was reading up on flat design for whatever reason and thought - "OK how can I just simplify this thing so that I reach Minimum Viable Product." So here's my MVP:
- Scrape 100 quotes from a website using BeautifulSoup.
- Store those quotes in a dictionary with two keys "quote" and "author".
- Choose a color palette form colorhunt.co.
- Put the quotes over the color palette. Save as 100 .pngs
- Start putting them up on IG and see what happens.
Some extra background. It's a little upsetting the amount of accounts that post motivational quotes and #entrepreneurshipfacts and even more upsetting the amount of people that follow these. I guess motivation is an undying need for us all and we'll consume it any way any time.
I started with #3 at colorhunt.co. To remove decision fatigue I just filtered by popularity and told myself to use one of the top three. I mean the whole point of the project is to appeal to the masses and not necessarily myself.
THEME_COLORS = ["#6a2c70", "#b83b5e", "#f08a5d", "#f9ed69"]
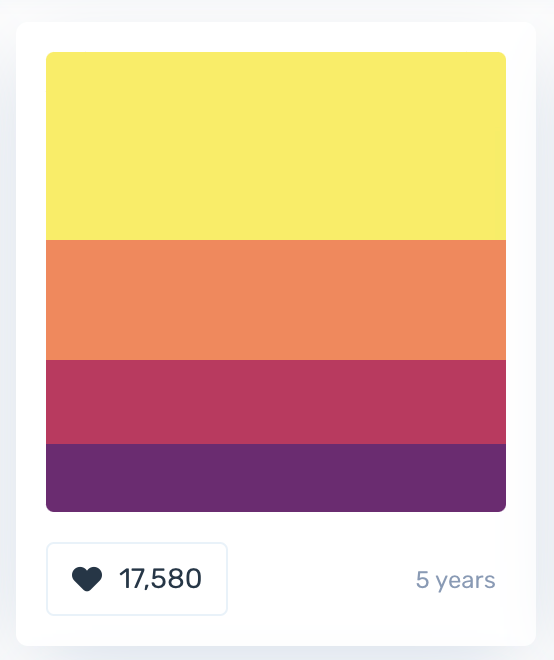
Next I googled motivational quotes. I ended up on a list from Forbes but ultimately got blocked form that page for accessing it too many times in a row when I was testing my BeautifulSoup code. Whoops.
I moved on and landed at https://www.positivityblog.com/success-quotes/
I wish I had taken some time to read the quotes. They aren't very good 😂 .
😑😑😑😑😑😑😑
“The distance between insanity and genius is measured only by success.”
– Bruce Feirstein
😑😑😑😑😑😑😑
“Life's real failure is when you do not realize how close you were to success when you gave up.”
– Unknown
It's OK It's OK. On page 1 of google results for a reason. I only took some time to read through them and got upset after finishing the project so by that moment it was too late. Probably for the best.
def pull_quotes_list_from_website():
response = requests.get("https://www.positivityblog.com/success-quotes/")
quotes_page = response.text
soup = BeautifulSoup(quotes_page, "html.parser")
all_quotes = soup.find_all(name="p")
with open("quotes.txt", mode="w") as file:
for quote in all_quotes:
file.write(f"{quote.text}\n")
def generate_quote_items(file_path):
items =[]
with open(file_path) as file:
while True:
quote = file.readline()
author = file.readline()
if not quote or not author:
break
items.append({'quote': quote, 'author': author})
return items
NOTE: Instagram shut down their API to be able to post using python (Instapy) or via the web (Selenium) so automating the posts was not an option. I almost trashed the project since I couldn't figure out how to automate it 100% but I pressed on.
All that was left was to make 100 images by combining the quotes and the text. In my infinite aesthetic imagine my mind's eye saw the following:




"OK Keegan put the quote in the middle and the author at the bottom."
So original. Probably shouldn't be bashing the quotes like I did and instead bash myself.
But the point is to NOT BE ORIGINAL. I pressed on.
def generate_quote_image(q_i, bg_color, days):
w = 500
h = 500
day = datetime.now() + timedelta(days=days)
day = day.strftime("%Y%m%d")
quote_font = ImageFont.truetype("Georgia Italic", 30)
author_font = ImageFont.truetype("Georgia", 15)
quote_text = "\n".join(textwrap.wrap(q_i['quote'], 30))
quote_author = q_i['author']
image = Image.new("RGB", (w, h), bg_color)
draw = ImageDraw.Draw(image)
draw.text(
(w/2, h/2),
text=quote_text,
fill="black",
anchor="mm",
align="center",
font=quote_font)
draw.text(
(w, h),
text=quote_author,
fill="black",
anchor="rm",
align="right",
font=author_font
)
#image.show()
image.save(f"images/{day}.png")
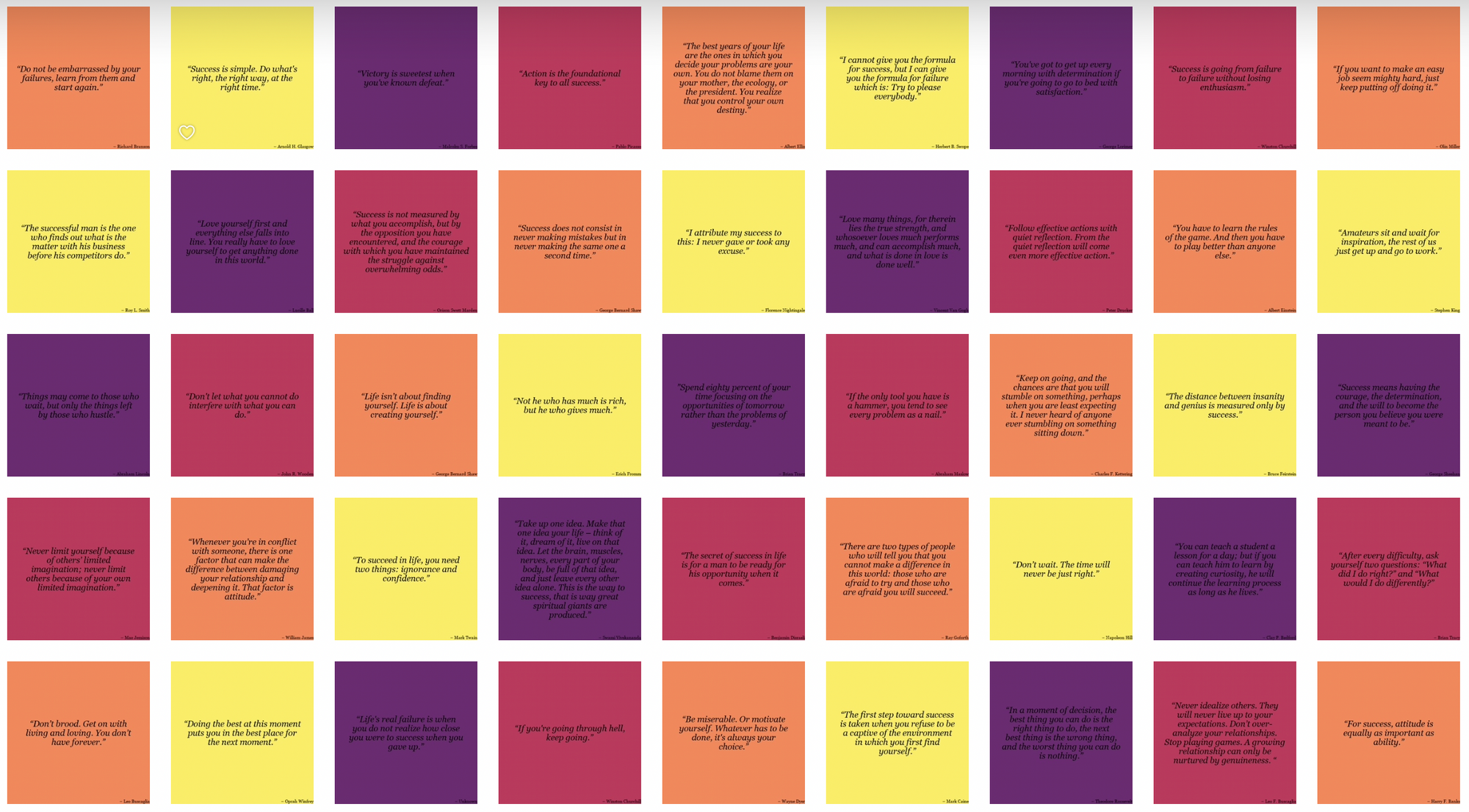
BOOM. I had made something. I had 100 .pngs on the hard drive and all that's left to do is post daily-ish and see what happens. I stole the hashtags from one of popular quotes accounts and stored it in a note to copy paste each time I post. I wonder how many followers 100 motivational quotes with fancy colors will get?
#motivation #inspiration #inspirational #motivational #quote #quotes #love #motivationmonday #happy #determination #inspire #qotd #positive #positivity #positivevibes #motivationalquotes #quoteoftheday #poetry #instaquote #quotestagram #loveyourself #entrepreneur #business